Ceil Function Matlab
Ismembc – Undocumented Helper Function
This function is used in stock Matlab ismember and setxor functions for quick processing of basic ismember functionality in "normal" cases: arrays of sorted, non-sparse, non-NaN data where only logical membership information is of interest to us . (not the found member index locations). Let's compare:>> % Initial Setup >> n=2e6; a=ceiling(n*rand(n,1)); b=ceiling(n*rand(n,1)); >> % Run ismember multiple times, to exclude JIT compilation overhead >> tic;ismember(a,b);toc; The elapsed time is 2.882907 seconds. >> tick;ismember(a,b);toc; The elapsed time is 2.818318 seconds. >> tick;ismember(a,b);toc; The elapsed time is 3.005967 seconds. >> % Now use ismembc: >> tick;ismembc(a,b);toc; The elapsed time is 0.162108 seconds. >> tick;ismembc(a,b);toc; The elapsed time is 0.204108 seconds. >> tick;ismembc(a,b);toc; The elapsed time is 0.156963 seconds.
Rounding
Roundfloat ceil (float value) float floor (float value) float round (float value [, int precision])
It's common that you want less precision than PHP gives you, in which case you should use one of PHP's selection of rounding functions: ceil(), floor(), and round(). Ceil() takes the number and rounds it to the nearest integer above its current value, while floor() rounds it to the nearest integer below its current value. Here is an example:
After running this code, $ceiled will be 5 and $floored will be 4. Author's note: The floor() function converts a floating-point number to an integer in much the same way as casting, except that casting is faster. The only difference is with negative numbers, where floor() will round -4.5 to -5 while the cast will return -4. The other function available is round(), which takes two parameters - the number to round and the number of decimal places to round.
As you can see, 4.5 is rounded to 5, while 4.4999 is rounded to 4. Well, the actual result of 1000/160 is 6.25 - you need six and a quarter coaches to carry 1000 people, and you'll only have ordered six because round() rounded up to 6 rather than 7 because it was closer. Tweet Next chapter: Randomization >> Previous chapter: Math Jump to: Functions Functions overview How to read function prototypes Working with variables Controlling script execution Working with date and time Read current time Convert from string Convert to string Convert from components Math Rounding Randomization Trigonometric conversion Other math conversion functions Basic conversion Math constants Playing with strings Reading part of a string Replacing parts of a string Convert to and from ASCII Measure strings Find a string within a string Return the first occurrence of a string Trim white spaces Surround your lines Change the case of strings Create a secure data hash Alternate data hash Auto-escape strings Printing pretty numbers Removing HTML code from a string ne Comparing Strings Padding a String Printing Complex Strings Parsing a String into Variables Basic Regex Regular Expressions with preg_match() and preg_match_all() Novice Regular Expressions Advanced Regular Expressions Guru Regular Expressions Regular Expression Replacements Syntax Examples regular expressions A regular expression helper Check if a function is available Extension functions Pause execution of a script Execute external programs Connection-related functions Modify the execution environment User functions Return values Parameters Pass by reference Return by reference Default parameters Number of variable parameters Variable scope in functions Priority scope with the GLOBALS array Recursive functions Variable functions Callback functions The declare() function and ticks Handling of non-English characters Undocumented functions Exercises summary Additional Reading Next Chapter Contents: Table of Contents
Copyright ©2015 Paul Hudson.
Name Already In Use
Many Git commands accept both tag and branch names. Creating this branch may therefore cause unexpected behavior.# Video | Ceil Function Matlab
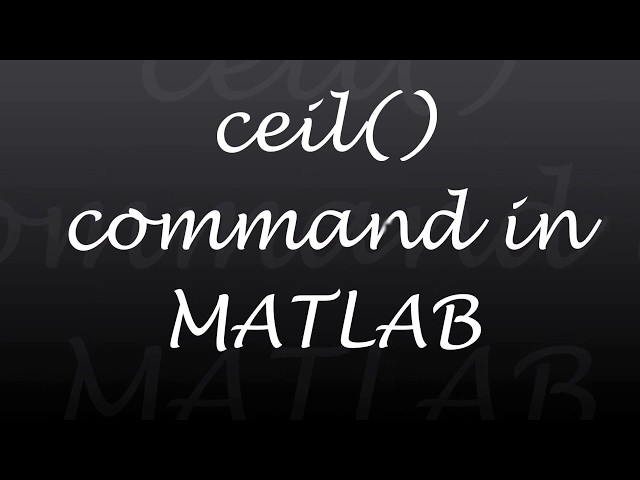
- linkedin-skill-assessments-quizzes/matlab/matlab-quiz.md
- Python’s Built-in round() Function
- Start Your First Python Project in 3 Steps: Grab Your Free Online Course Right Now!
Ceil Function Matlab Example
Ceil: Integer Functions (Matlab Style)
ceiling()ceiling()
not
is an alias for and rounds to the smallest integer equal to or greater than
Fix() truncates values to 0 and is an alias for trunc() .
Ceil Matlab In Python
Round Matlab Function: Floor, Ceil, Fix And Round
More specifically, you will learn to:Round to the nearest integer using the round function
Round using the floor and fix functions
Round using the ceiling function
Round to X decimal places
Round to nearest integer: rounding function
Round to nearest integer for positive and negative values: If you want to round a number to its nearest integer value in MATLAB, you must use the round function. In short, this will round up to a positive value:
Similarly, it will also be rounded for a negative value:
Round to X decimal places and any nearest desired value
Round to X decimal places: you can round to X decimal places with the following code: decimal = 3; value = round ( 10 ^decimal*value ) / 10 ^decimal; For example, to round to 3 decimal places, you just need to set the decimal variable to 3, and to round to 4 decimal places, run the code above for decimal=4. For example, suppose you want to round to the nearest quarter: nearest value = 0.25; value = round(value/nearestValue) * nearestValue; Finally, it would be:
Key points to remember:
To round in MATLAB, use: floor to round down to a whole number:
4.6 -> 4 -4.6 -> -5
correction for rounding towards 0:
4.6 -> 4 -4.6 -> -4 To round in MATLAB, use this:
-4.3 -> -4 -4.6 -> -4
To round to the nearest integer, use round:
4.3 -> 4 4.6 -> 5
To round to X decimal places, use:
value = round ( 10 ^decimal*value ) / 10 ^decimal; To round to the nearest desired value, use: value = round ( value/nearestValue ) *nearestValue;
If you liked this article, here are some other articles you might also like:
If you want to know more about the practical tips that helped me stop wasting time doing mindless work (like working with Excel files and writing reports), I wrote a little reference book about it. topic :
Ceil Built-In Function Matlab
Weekly Tutorial Exercise
The last two digits of \( 2020^{2020}\) can be found more efficiently than in class by using a binary representation of the power \(2020\). From the binary representation \[ 2020 = (11111100100)_2 = 2^{10} + 2^{9} + 2^{8} + 2^{7} + 2^{6} + 2^{5} + 2 ^{2} \] the number \(2020^{2020}\) can be expressed as \[ 2020^{2020} = {2020}^{2^{10}} \cdot {2020}^{2^{ 9}} \cdots {2020}^{2^5}\cdot {2020}^{2^2} \] %% Find the last two digits of 2020^2020 using the power algorithm for modular arithmetic n=2020; bin_dig = dec2bin(n); % the binary, represented by the characters "1" and "0" (not numbers!) pow2k = [mod(n,100)]; % a vector of 2020(2^k) (mod 100), start with k=0 for k=1:length(bin_dig) % add the last two digits of 2020^(2^k) in the vector end last_two_dig = 1 ; for k=1:length(bin_dig) if (bin_dig(end+1-k)=='1') % if the binary digit is '1', multiply the factor 2020^(2^k), starting from the highest small power last_two_dig = % complete this line, to update the last two digits end end last_two_dig % displays the last two digitsCollatz conjecture. Consider the following operation on an arbitrary positive integer from \(n\) to \(f(n)\) \[ n \mapsto f(n)=\begin{cases} 3n+1, &\mbox{if } n \mbox{ is odd},\cr n/2, & \mbox{if } n \mbox{ is even}. counter function = collatz(N) %% For the positive integer in input N, return the number of steps, such that "1" appears in first counter = 0; % the initial value n = N; while (n) ~= 1) % completes this part, performs one step of the iteration over n, and increases the counter by one end You can test your function, for the following values: (1) the output of collatz (1) should be 0; (2) the output of collatz(2) should be 1; (3) the output of collatz(3) should be 7 . Write a loop to find all integers \(n\) from \(2\) to \(10\), such as \( \lfloor (n^3)^{\frac{1}{3}}\rfloor \ ) returns \(n-1\) instead of the expected result \(n\). Write a loop to find all integers \(n\) from \(2\) to \(10\), such as \( \lceil (n^5)^{\frac{1}{5}}\rceil \ ) returns \(n+1\) instead of the expected result \(n\). Find these three solutions using a loop for \(n\) taking values from \(1\) to \(17\) (MATLAB can't handle larger integers like \(18!\), and you'll have to maybe use a symbolic integer which will be covered next week).
# Images | Ceil Function Matlab
Name already in use
How to Round Numbers in Python